Using jQuery with µWeb¶
Introduction¶
One way to improve look and feel of your user interface of your µWeb application is to use JavaScript to dynamically script the HTML of your pages. jQuery is a cross-browser JavaScript library designed to simplify the client-side scripting of HTML. jQuery is the most popular JavaScript library in use today. You can augment your µWeb application very easily with jQuery because µWeb ensures you can arbitrarily add attributes and explicitly manipulate your HTML output in almost any desired manner, if you desire to do so.
The following is a small example of how to apply jQuery to a µWeb application in order to provide html styled help tooltip popups on µWeb web pages.
What we want to do¶
µWeb provides page and element level help keywords. E.g. we may have a simple button defined as so:
1 2 3 4 5 6 | field enable {
label "Enable SNMP"
type boolean
default 0
help {Enable or disable the SNMP agent.}
}
|
This help text on line 5 may be displayed by the page however it likes, e.g. it may be presented in edit mode directly on the page as ordinary text. However, we wish to display it with embedded HTML styling on a tooltip, i.e. dynamically display the help text beside the field as the mouse rolls over the field.
We redefine the help test as follows, to include HTML styling:
1 2 3 4 5 6 7 8 9 10 | field enable {
label "Enable SNMP"
type boolean
default 0
help {
Enable or disable the SNMP agent.
<br>
<b>Note:</b> The SNMP agent supports SNMPv1 and SNMPv2c
}
}
|
Then we want to to present that help within an enhanced tooltip like the following:
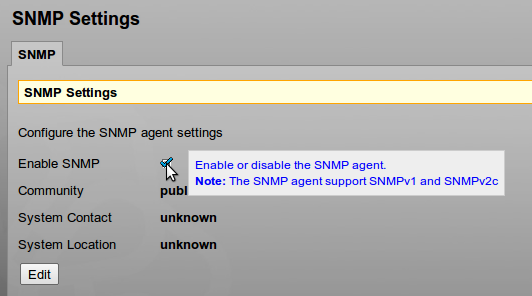
We will also add similar tooltips to the other fields on the SNMP page.
The way we will implement this function is to add help text as a title attribute on each displayed element in the HTML and use a jQuery function to extract and output that text as the mouse rolls over the element.
Additions to the .app file¶
The first step is to add the jQuery library to our app. We also add our own file containing the jQuery code we will create. Here is the modified app file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | appname "Simple Demo"
defaultpage snmp
toolbar tcl
stylesheet /css/basic.css
icon /img/uweb-logo.ico
stylesheet id=theme /css/snmpdemo.css
javascript http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.js
javascript /javascript/jquery.tooltips.js
pages {
*.page
}
# vim:se ts=4:
|
Note the addition of the jQuery library at line 10 and the addition of our own tooltip jQuery code at line 11. We are using the Google hosted CDN version of jQuery here but you can of course host a local copy on your server.
Changes to the help definitions in the page¶
We need to output the help text for each field in the HTML output. µWeb provides this as a standard feature by adding the -title attribute to the help keyword as in the following example. If you add the -title attribute to the page keyword then it is assumed that all field help keywords are to have that attribute also. You can also choose just to add the -title attribute to specific fields.
help -title "Configure the SNMP agent settings"
Adding the jQuery code¶
The final step is the add the actual jQuery code where all the work is done.
jquery.tooltips.js:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | /* Jquery function to display a tooltip */
$(function() {
$('.elemvalue')
.each(function() {
$(this)
.after($('<span/>')
.attr('class', 'tooltip')
.html($(this).attr('title')))
$(this)
.removeAttr('title')
})
.hover(function() {
var text = $(this).next('.tooltip');
if (text.attr('class') == 'tooltip') {
text.toggle()
.css('top', text.parent().position().top - 3)
.css('left', e.PageX + 20);
}
return false;
})
});
/* vim: se ts=4: */
|
This code does the following:
- Line 3:
Declares a function which is called by jQuery after the HTML document is loaded.
- Line 4-5:
Calls a jQuery each() function to iterate over all µWeb element values and then adds a new tooltip class element after all span elements. The content of the tooltip element is filled with original title attibute. The original title element is cleared to avoid the browser outputting a standard tooltip.
- Line 13:
Also adds a jQuery hover() function to toggle the display of the HTML title (using the text of the next class tooltip element) to the screen.
Final completed µWeb page¶
Here is the final completed µWeb page including all the help definitions which uses the above jQuery code.
snmp.page:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | title "SNMP Settings"
label "SNMP"
help -title "Configure the SNMP agent settings"
bar "SNMP Settings"
init {
package require common
}
text {
display display_page_help
}
field enable {
label "Enable SNMP"
type boolean
default 0
help {
Enable or disable the SNMP agent.
<br>
<b>Note:</b> The SNMP agent support SNMPv1 and SNMPv2c
}
}
field community {
label "Community"
type text
default public
help {The <i>(read-only)</i> SNMP community string}
}
field syscontact {
label "System Contact"
type text
default unknown
help {The value to return for mib-2 sysContact.0}
}
field syslocation {
label "System Location"
type text
default unknown
help {The value to return for mib-2 sysLocation.0}
}
button submit {
label Submit
stdmode none
submit {
if {[cgi save]} {
# Something was changed
# start/stop/HUP snmpd as appropriate
cgi success "SNMP Settings Updated"
}
}
}
button edit {
label Edit
editmode none
setmode edit
help "This is the help button"
}
button cancel {
label Cancel
stdmode none
}
# vim:se ts=4:
|